On the second day of the Microsoft Build conference, during the Keynote, Microsoft had some awesome announcements related to Mobile development. In a following session James Montemagno and Miguel De Icaza got into more detail and even showed more epic new features. As always to two put on a great show while presenting!
Together with my colleague Marco, I’ll cover announcements made at the Microsoft Build conference in a series of blogposts. You can also read these posts on his blog.
Xamarin Live Player
When Xamarin Live Player (XLP) was announced during the Build keynote, most of the audience was pleasantly surprised. Until now developing on Windows for iOS was a serious hassle because it required a Mac to compile the app. This setup works well when you have an expensive Mac and a fast and reliable network, but in a lot of scenarios these builds take up a lot of time.
With XLP you don’t need a Mac build host anymore! You just install the Xamarin Live Player app from the App Store or Play Store, pair your device in Visual Studio and boom! You’re ready to debug on the device. Just select your device (annotated with “Player”) en hit run:
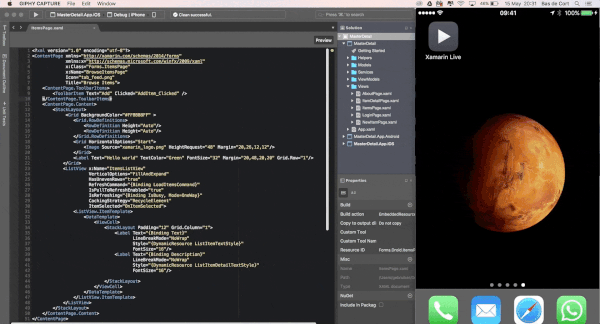
Besides the build improvements XLP has more to offer! You can also modify your Xamarin.Forms or Xamarin.Classic app while debugging so you can easily test changes to your app without stopping and restarting the debug process.
This will really speed up the development! To achieve this you first have to open the view you want to debug live. For now a Xamarin.Forms Xaml and iOS storyboards are supported, when XLP becomes GA all types of views should be supported. After opening your view file, you can run the with Visual Studio by clicking “Run -> Live Run Current View” on Mac or “Tools -> Xamarin Live Player -> Live Run Current View” on Windows.
XLP is still in preview and after some testing we are still experiencing a few difficulties. If you want to give XLP a try, you’re probably best of using the examples provided by James Montemagno.
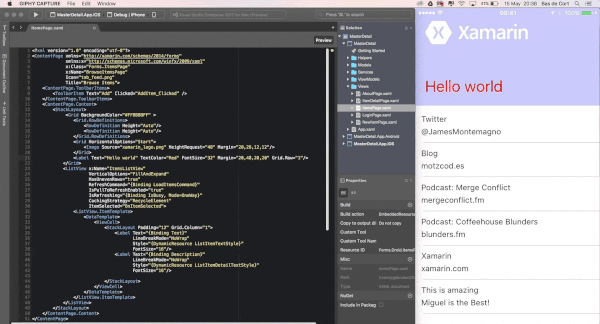
Fastlane
If you’ve ever developed an app for iOS you probably felt the pain of having to provision your app in the Apple Developer Portal. Although there is a good (and very long) read on how to accomplish this, you don’t want to waste any time on this. This is where Fastlane comes to the rescue. With Fastlane tools installed you are able to provision your app with a few clicks in Visual Studio! Fastlane also comes in handy when releasing your app to the Stores. It handles all tedious tasks, like generating screenshots, dealing with code signing, and releasing your application.
Xaml standard 1.0
During the Build Conference Microsoft also introduced a new standard for XAML, called XAML Standard 1.0. The standard will describe a specification for cross-platform user interfaces for both UWP and Xamarin.Forms. As for now, XAML Standard is in a very early stage and Microsoft encourages developers to contribute to their specification. 1.0 should become available later this year.
Bindings simplified
Xamarin was already supporting usage of native libraries with Android binding and iOS binding projects. Because of the complex nature of binding projects, Xamarin released the CocoaPods Importer which will simplify importing CocoaPods with a few clicks. With this importer you can easily transform a CocoaPods library to a .NET library.
iOS Extensions
When you’ve created extensions for your app, these extensions will run in a separate process. Therefor the Xamarin runtime was included for every extension. With the latest version of Xamarin, the runtime is only included once for all your extensions. This will result in a smaller app size and improved performance. The latest version of Visual Studio also allows multi-process debugging, that will come in handy when debugging your app combined with your extensions.

AOT for Mac and Android, Hybrid mode
The compilation process of Xamarin.Android and Xamarin.iOS apps was quite different until now. One big difference was that Android was compiled Just In Time, iOS was compiled Ahead Of Time. After the compilation of iOS apps, the LVVM optimizes the generated code to get high performance. Xamarin announced to also start supporting AOT for Android and Mac apps, that will result in better performance. When you compile AOT you might lose some flexibility (for example: no dynamic code loading), and therefor Xamarin also introduced an Hybrid mode. The Hybrid mode will work for platforms that support AOT as well as JIT. It will compile AOT, but also add the bits needed for JIT.

Embedinator-4000
While the Xamarin platform mostly focuses on writing code in C# and compiling this to a native application, the Embedinator takes a bit of a different approach. The Embedinator makes it possible to write your code in C# and then compile this into a framework library for Java, Swift, Objective-C or C/C++. Therefor you can easily share your C# code with truly native apps. Miguel De Icaza promised that more languages will be added. As for now, the Embedinator can be used from command line, in the future this will be integrated in Visual Studio.
SkiaSharp
SkiaSharp is a cross-platform 2D graphics API for .NET platforms based on Google’s Skia Graphics Library (which is used by apps like Google Chrome). Xamarin announced that it will be supporting Android, iOS, Mac, UWP, tvOS and Windows. Also support for SVG files is added in the latest release. James showed an awesome demo of The Kimono designer which is build on top of SkiaSharp.
Xamarin.IoT
The latest alpha of Xamarin also contains support for Linux based IoT devices! It will add a new project template for Xamarin.IoT and allow you to deploy and debug your IoT app remotely. Debugging will work similar to debugging Xamarin applications. The IoT Hub SDK will make it easy to communicate with your Azure IoT Hub. The library supports communication with MQTT and AMQP message queues.
Mobile Center
Microsoft is putting a lot of effort in making Mobile Center a full fletched CI/CD solution. With the latest release they added support for UWP and the ability to send push notifications to Android or iOS applications. Mobile Center is still in preview, but is expected to become GA later this year.
Project Rome for iOS
Last year Microsoft announced Project Rome SDK for Android. This year at the Build conference Microsoft also announced the iOS SDK. Project Rome creates a bridge between the different platforms for a seamless user experience. The SDK allows developers to start and control apps over platforms and devices.
If you have any comments or want to share your experience, don’t hesitate to contact me on Twitter.
Related links: